Instruction Set Architectures
Instruction Set Architectures: The Language of Computers
To perform any task on a computer, three essential components are required: the computer itself, data, and a program. A computer program is a sequence of instructions that tells the computer how to process data to achieve a specific goal. These instructions are the building blocks of all computational tasks, and understanding them is key to understanding how computers work.
Programs, Instructions, and Instruction Sets
At the heart of every computer program are instructions, which are the smallest units of work a computer can perform. Each instruction is a command that tells the computer to perform a specific operation, such as adding two numbers or moving data from one location to another.
The collection of all instructions that a computer can execute is known as its Instruction Set. Think of the instruction set as the computer's vocabulary—the set of words it understands. Just as human languages have dialects, different computer architectures have different instruction sets. However, most instruction sets share common features, such as basic operations like addition, subtraction, and jumping to different parts of a program. Once you understand one instruction set, learning others becomes much easier.
The Anatomy of an Instruction
Every instruction consists of several key elements:
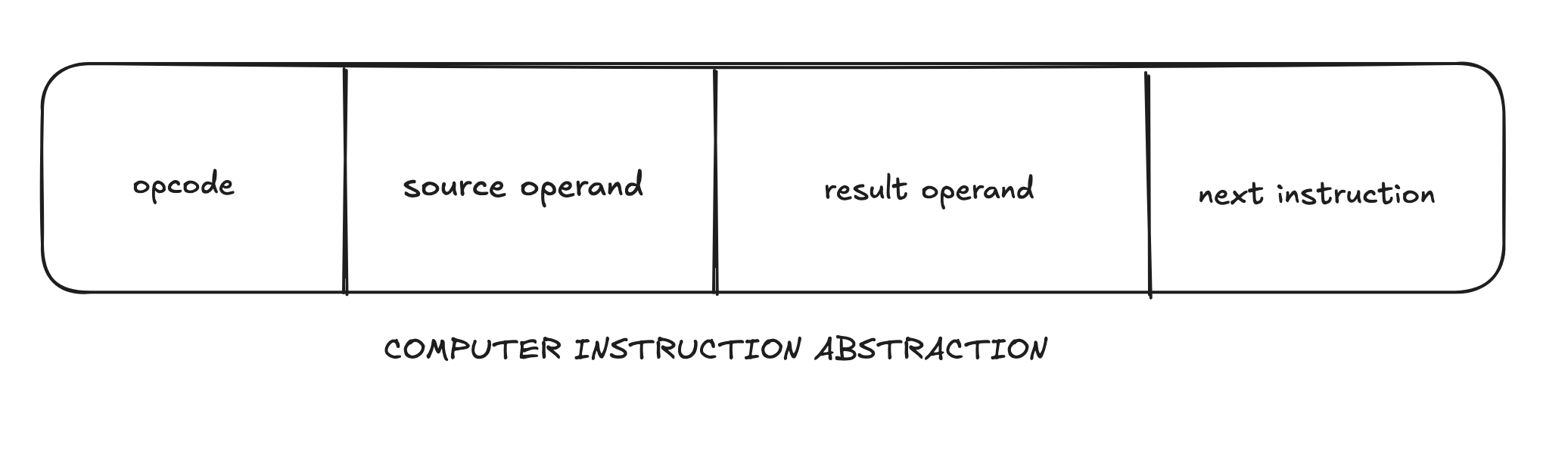
- Opcode (Operation Code): Specifies the operation to be performed (e.g., add, subtract, load, store).
- Source Operand(s): The input(s) for the operation. An instruction can have one or more source operands.
- Result Operand: The location where the result of the operation will be stored.
- Next Instruction Reference: Tells the processor where to fetch the next instruction.
These elements work together to define what the computer should do at each step of a program.
Operands: Where Data Resides
Instructions operate on operands, which are the data values being processed. Operands can be stored in several places:
- Registers: Small, fast storage locations within the CPU. Registers are used to hold data that is being actively processed.
- Memory: A larger but slower storage area where data is stored when not in use.
- Constants: Fixed values that are embedded directly within the instruction itself.
For example, consider the following high-level code and its corresponding MIPS assembly code:
In this example, a
, b
, and c
are operands. The add
instruction tells the computer to add the values in b
and c
and store the result in a
. The operands can be stored in registers, memory, or as constants within the instruction.
The MIPS Register File
The MIPS architecture uses 32 registers, collectively known as the register file. Registers are incredibly fast, but they are limited in number. This is why programs also use memory to store data. By combining registers and memory, a program can access a large amount of data relatively quickly.
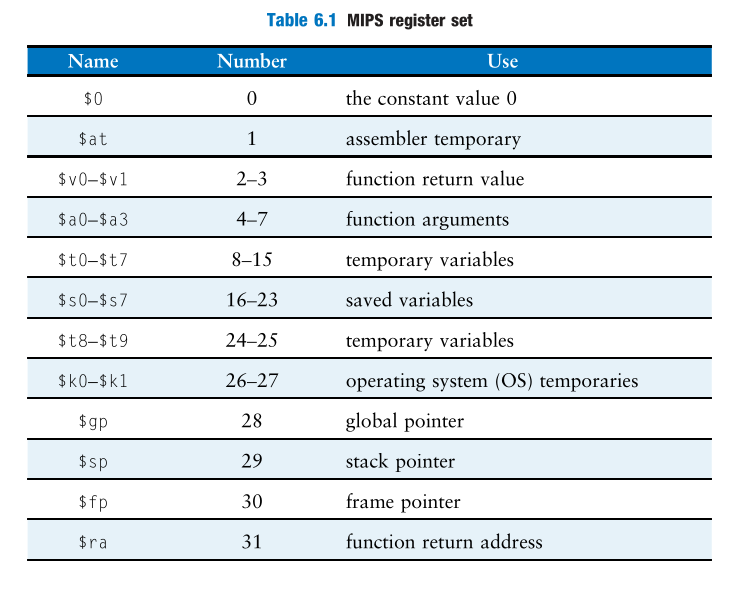
Memory: The Larger Storage
While registers are fast, they are limited in number. Memory, on the other hand, can store vast amounts of data but is slower to access. MIPS uses a byte-addressable memory, meaning each byte in memory has a unique address. This allows for fine-grained control over data storage and retrieval.
Word vs. Byte Addressable Memory
-
Word Addressable Memory: In this model, each word (typically 32 bits) has a unique address. For example, the word
0xF2F1AC07
might be stored at address00000001
. -
Byte Addressable Memory: In MIPS, memory is byte-addressable, meaning each byte has a unique address. Words are typically 4 bytes long, so the address of a word is four times its word number.
For example, the following MIPS assembly code reads and writes words from memory:
We do a lot of memory addressing with offsets and a base address by orders of 4. We can use the decimal or hexadecimal for the offset value.
Constants and Immediate Operands. The immediate specified in an instruction is a 16-bit two's comple-ment number in the range . Which is in terms of bit size width.
Instruction Formats: R-type, I-type, and J-type
MIPS instructions come in three formats, each designed for different types of operations:
-
R-type (Register-type): Used for operations that involve three registers. The instruction format includes fields for the opcode, source registers, destination register, and a shift amount.
- Opcode: Determines the operation (e.g., add, subtract).
- Source Registers (rs, rt): The registers containing the operands.
- Destination Register (rd): The register where the result will be stored.
- Shift Amount (shamt): Used in shift operations to specify the number of bits to shift.
-
I-type (Immediate-type): Used for operations that involve two registers and an immediate value. The immediate value is a 16-bit constant embedded in the instruction.
- Opcode: Determines the operation (e.g., add immediate, load word).
- Source Register (rs): The register containing the first operand.
- Destination Register (rt): The register where the result will be stored.
- Immediate (imm): A 16-bit constant used in the operation.
-
J-type (Jump-type): Used for jump instructions that change the program counter to a new address. The instruction contains a 26-bit address field.
Connecting the Dots: How It All Fits Together
Understanding instruction sets, operands, and memory is crucial for understanding how a computer executes programs. Here's how everything connects:
- Program Execution: A program is a sequence of instructions stored in memory. The CPU fetches each instruction, decodes it, and executes it.
- Operand Access: During execution, the CPU accesses operands from registers, memory, or constants embedded in the instruction.
- Instruction Formats: The format of an instruction (R-type, I-type, J-type) determines how the CPU interprets it and what operations it performs.
- Memory Hierarchy: Registers provide fast access to data, while memory offers larger storage capacity. Programs use both to balance speed and storage needs.
Instruction Set Architectures are the foundation of how computers execute programs. By understanding the elements of instructions, the role of operands, and the different instruction formats, you gain insight into the inner workings of a computer. Whether you're working with MIPS or another architecture, these concepts are universal and provide a solid foundation for further exploration in computer systems.